Stacks - Learn by visualizing
Stack works on the basis LIFO or FILO principal.
A Stack is a linear data structure that stores an ordered sequence of elements based on the LIFO (Last-In-First-Out) principle. This means that the last element inserted into the stack is the first one to be removed. It is also referred to as the FILO (First-In-Last-Out) principle, where the element that was inserted first is the one that will be removed last.
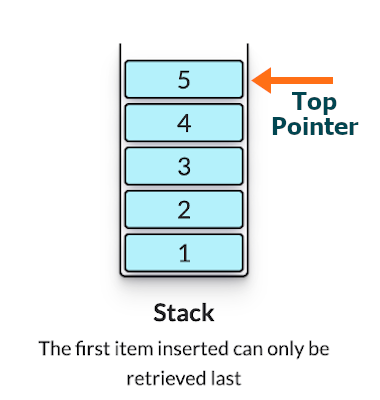
As shown in the diagram, a stack has only one open end, and elements can only be accessed from the top. To implement a stack, you only need a top pointer that points to the top-most element in the stack. Sometimes, a limit is imposed on the number of elements that can be added to the stack. If you attempt to insert more elements than the allowed limit, a condition known as stack overflow occurs. Have you ever wondered how Stackoverflow got its name?
Stack Operations
There are two main operations that can be performed on the stack:
- Push: Insert an element onto the top of the stack.
- Pop: Remove the top-most element from the stack.
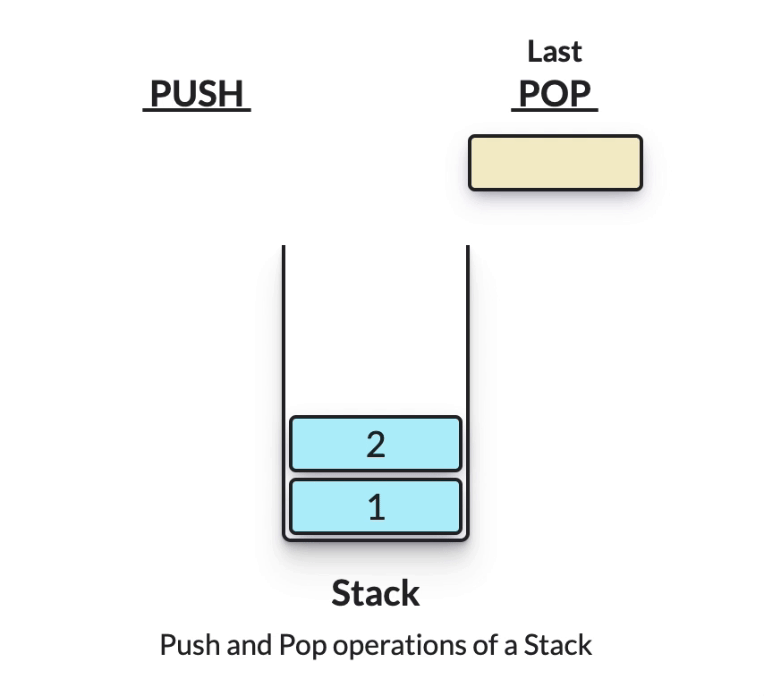
There are other operations like Peek, which is to retrieve the top most element of the stack without deleting it. You can also perform other operations like to check if the stack is empty or full.
Stacks are commonly used in expression evaluation, parenthesis matching, and syntax parsing, which are widely used in calculator programs. Additionally, stacks can be utilized to implement efficient backtracking algorithms.
Questions related to stacks are frequently asked in technical interviews and coding challenges. Below, I’ve implemented solutions to some of these problems, along with animations to help visualize the algorithms and enhance your understanding.
Stack Problems
Take a look at some of these stack problems and their visualizations!
- Design Browser History
- Check for valid parenthesis
- Find the index of closing bracket for a given opening bracket
- Find the lexicographically smallest substring with distinct characters
- Longest Valid Parentheses
- Find The Next Bigger Number
- Simplify Absolute UNIX Path
- Reverse a String Using Stack
- Sort A Given Stack
- Stock Span Problem